Vue Js Change Array Index Position by UP and Down Click:Vue.js is a JavaScript framework that provides a declarative way of building web applications. One of its core features is its reactivity system, which makes it easy to manipulate and update data in real-time.
To change the index position of an array in Vue.js by up and down click, you can use a combination of event listeners, methods, and computed properties.
Firstly, you can create two buttons for each item in the array, one for moving it up and another for moving it down. You can then add event listeners to these buttons that trigger methods to update the position of the item in the array.
These methods can make use of the splice
function to remove the item from its current position and insert it at the desired position. You can also make use of computed properties to display the updated array.
Overall, this approach allows you to easily manipulate the position of items in an array in Vue.js with just a few lines of code.
How can I implement a Vue.js feature that allows the user to change the position of an element in an array by clicking an “up” or “down” button?
This script uses Vue.js to create a list of programming languages and provides methods to move items up and down within the list. When an item is moved up, it is removed from its current position and inserted at the previous index. When an item is moved down, it is removed from its current position and inserted at the next index.
The methods
object defines two functions: moveUp
and moveDown
. These functions take an index as an argument, which corresponds to the index of the item that should be moved.
When moveUp
is called with an index, it first stores the value of the item at that index in a temporary variable. It then removes the item from the array using the splice()
method, starting at the index and removing one item. Finally, it inserts the stored value at the previous index using splice()
again, starting at the index minus one and inserting the stored value.
moveDown
works similarly, but inserts the stored value at the next index instead.
Overall, these functions allow the user to rearrange the order of the programming languages in the items
array by moving them up or down one position at a time. The reactive user interface provided by Vue.js ensures that the view is updated automatically whenever the data changes, making for a seamless user experience.
Vue Js Change Array Index Position by Up and Down by Click Example
<div id="app">
<ul>
<li v-for="(item, index) in items" :key="index">
{{ item }}
<button @click="moveUp(index)" :disabled="index === 0">Up</button>
<button @click="moveDown(index)" :disabled="index === items.length - 1">Down</button>
</li>
</ul>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data: {
items: ['Vue', 'React', 'Nuxt', 'PHP','Node']
},
methods: {
moveUp(index) {
const temp = this.items[index]
this.items.splice(index, 1)
this.items.splice(index - 1, 0, temp)
},
moveDown(index) {
const temp = this.items[index]
this.items.splice(index, 1)
this.items.splice(index + 1, 0, temp)
}
}
});
</script>
Output of above example
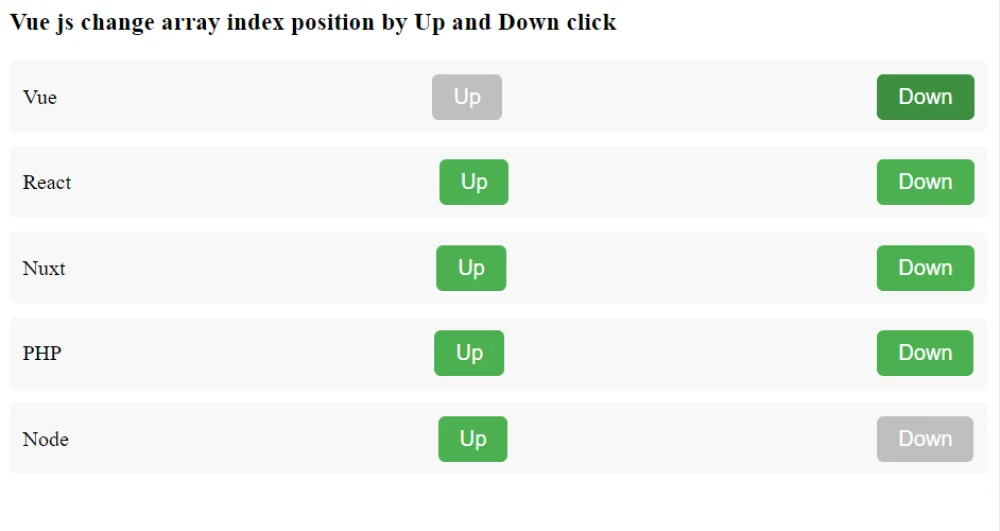